Table of contents
Non-Fungible Tokens (NFTs) are unique digital assets that can be stored on any blockchain, representing the ownership or proof of authenticity across a wide range of items including, but not limited to artwork, music, or even virtual real estate. Alongside the rise of NFTs, DALL-E by OpenAI has been introduced as a cutting-edge AI model capable of generating intricate and detailed images from a simple textual prompt. This allows us to incorporate the creativity of AI image generation, with blockchain technology creating new opportunities that were once deemed unimaginable.
This guide will walk us through the relatively simple process of creating our own NFTs using images generated through DALL-E. We will go over the basics of how to use DALL-E to generate images based on descriptions, and then take those generated images to be minted into NFTs using Original, a feature-rich blockchain platform. Whether you’re a digital creator looking to expand your portfolio or a Web 3 enthusiast curious about the intersection of AI and blockchain, this simple guide is just what you need to get started!
Creating Compelling Images with DALL-E 3
This section walks you through the DALLE-3 API, the nuances of image generation, and how we can customize our images according to our desired outcomes.
Accessing DALL-E 3
In order to start using DALL-E 3 for image generation, we first need to have access to an OpenAI API account first. We can do that by heading over to this OpenAI link and going through the sign-up process.
Upon logging into the OpenAI account, we’ll be redirected to the OpenAI API Developer Platform. From the sidebar, we will head on over to the API Keys page, and after verification, grab our API key and keep it in a safe place since we’ll be coming back to this shortly.
From there, we’ll go back to the dashboard, and then navigate to the QuickStart Guide, and select the programming language we’ll use for your application. This guide will focus on the Node.js implementation, however there are clear guides for curl and Python as well.
We offer a free audit of your web2 game
Click here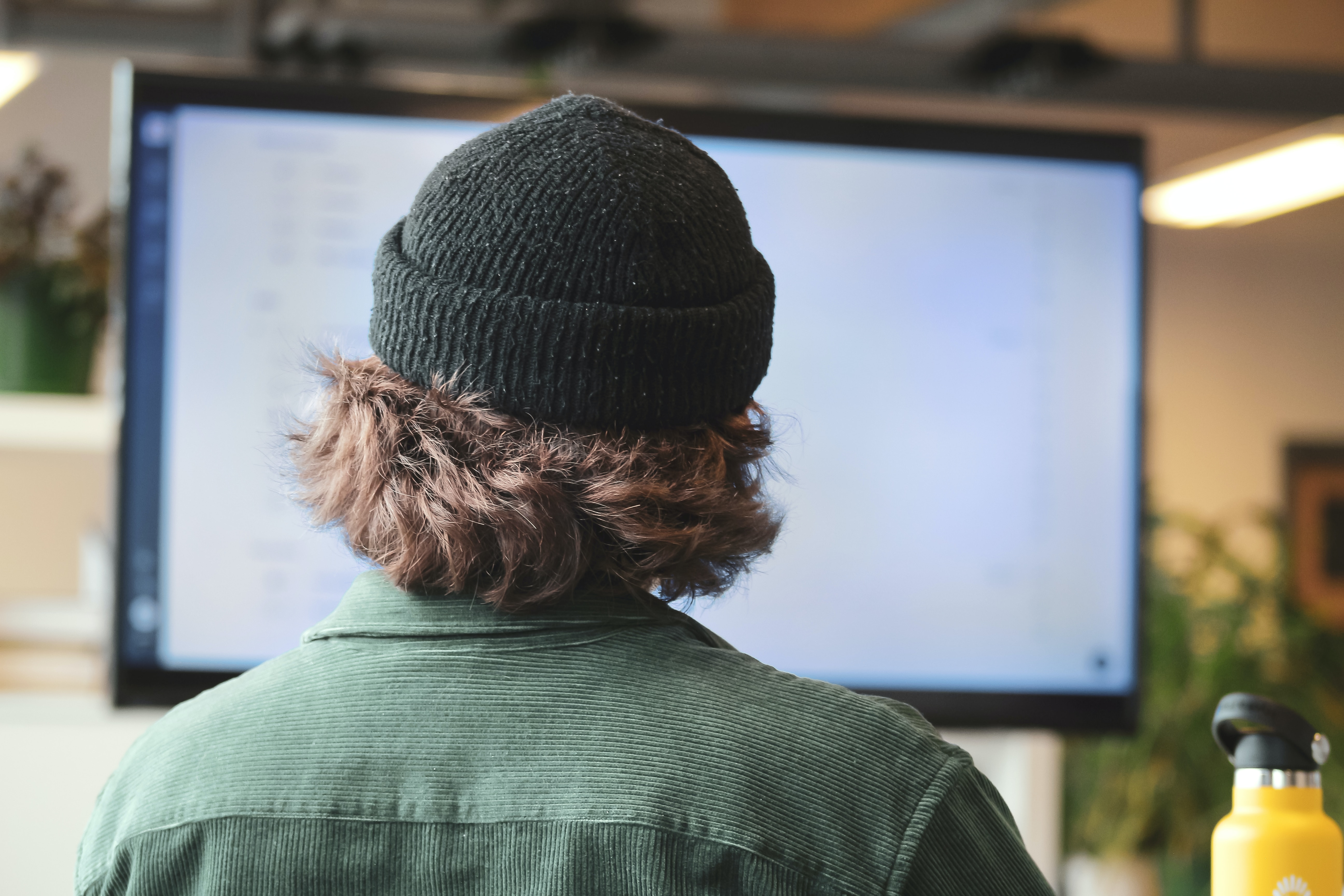
In our Node.js environment, we will proceed with the installation of the OpenAI Node.js library with the following command.
npm install --save openai
#or
yarn add openai
After the install is successful, we can use our API key to authenticate with OpenAI as follows.
import OpenAI from "openai";
const openai = new OpenAI({
apiKey: "PASTE_YOUR_OPENAI_API_KEY_HERE",
});
To keep things simple we will directly use our API key, though using them as environment variables would be ideal for production applications.
And we’re done! You have successfully set up a Node.js environment for using the OpenAI API. In the ensuing section, we will use this to create custom and unique images via DALL-E 3! Also, If this sounds like too much work, then fret not! This model is also directly accessible through ChatGPT Plus, a paid subscription that gives you access to DALL-E and ChatGPT-4, providing a seamless integration for generating images.
Making the API Call to Generate Images with DALL-E 3
After setting up our Node.js environment according to the OpenAI QuickStart Guide, we can dive into generating unique and realistic images with the DALL-E 3 Model. The Images API offers three methods for image manipulation. Our primary concern for NFT generation is with creating new images from text prompts, it may prove beneficial to your use case to note the extended capabilities of DALL-E.
DALL-E 3 is quite good at creating images from scratch based on text prompts, which is our main use case for the application. However, for those working with DALL-E 2, they can leverage extensive features which include editing pre-existing images by replacing specific areas with new content, and generating variations of an existing image.
const OpenAI = require("openai");
const openai = new OpenAI({
apiKey: "PASTE_YOUR_OPENAI_API_KEY_HERE",
});
async function main() {
const image = await openai.images.generate({
model: "dall-e-3",
prompt: "A very well written article",
});
console.log(image.data);
}
main();
In the response to this API request, you’ll get the image URL as well as the revised prompt in the following json body:
[
{
revised_prompt: ...,
url: ...
}
]
It is important to note that generated URLs expire after an hour. For a detailed reference on the DALL-E 3 API and how to use it according to your use case, refer to the API Reference Docs and Image Generation Docs
Minting Your DALL-E 3 Creations as NFTs
This wouldn’t be a simple article had Original not done all of the heavy lifting for us! With the Original Node.js SDK, we can easily use our existing Node.js environment initially set up for using DALL-E 3, in order to use those images to mint NFTs.
First Grab Your Free API Key From Original :
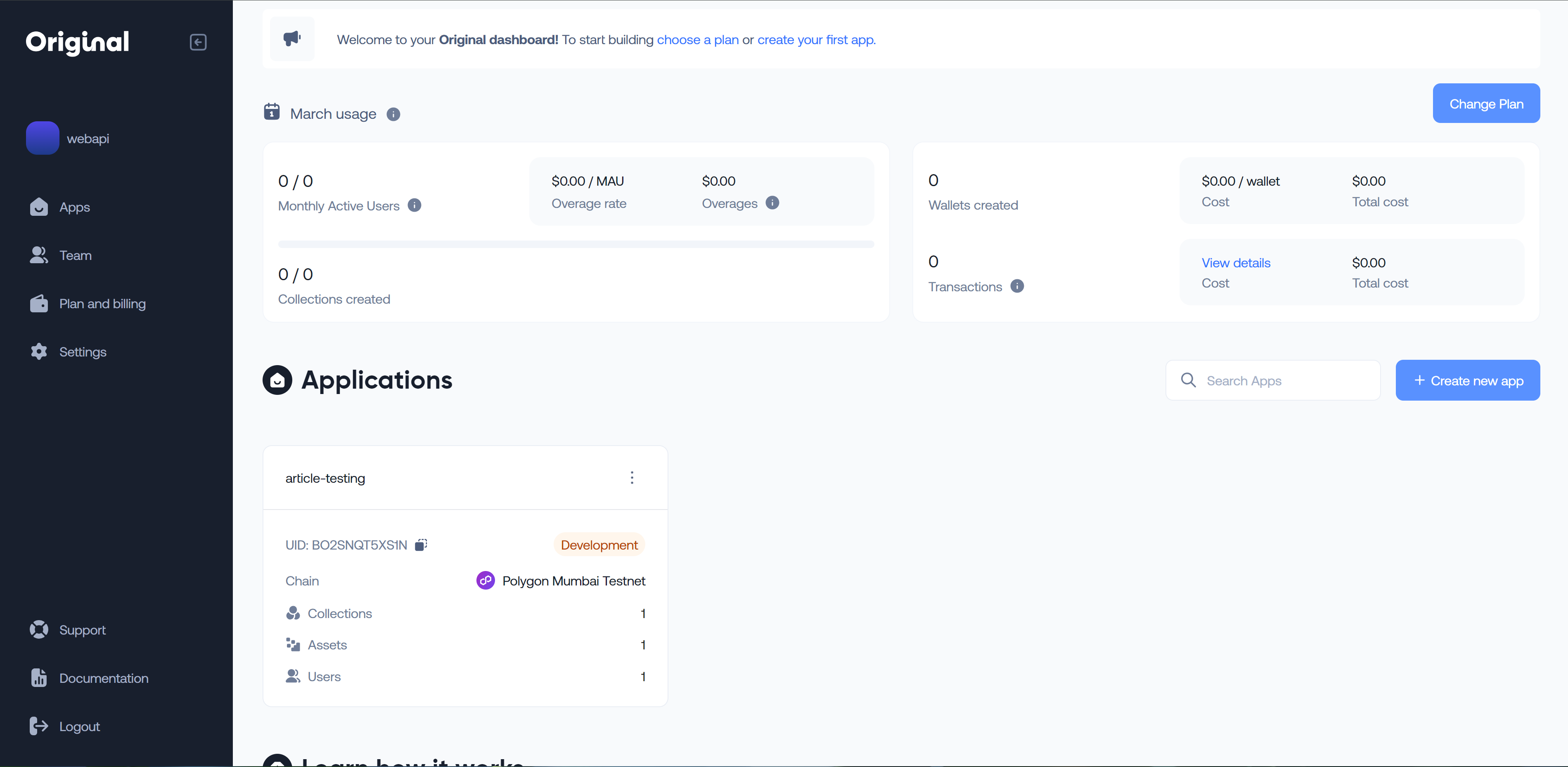
To get started, use the official QuickStart guide and set up the Original developer console by creating an account.
After you’ve created an account, on the Original Developer Console, navigate to the Apps menu, and click Create New App.
Follow the app creation process according to the create new app guide, and then proceed over to creating a collection. For this, you have to navigate to the app you just created, and on the app’s panel on the right, there will be an option to create a collection. For details on all of the collection specifications, refer to this guide.
After you have created your collection for the app, navigate back to the app panel, and click on the API Keys tab in the menubar. There, you can create new API and secret keys. With these, we’ll be able to access endpoints that enable us to mint our own NFTs using the unique images that we generated from DALL-E.
For this example, we will be using the Original Node.js SDK. You can add the SDK to your app by using the following npm command.
npm install --save original-sdk
Then in your Node.js application, use the SDK to import OriginalClient and set your API key and secret for the development environment, though using them from the environment variables is recommended:
const { OriginalClient, Environment } = require("original-sdk");
const client = new OriginalClient(
"YOUR_API_KEY",
"YOUR_SECRET_KEY",
{
env: Environment.Development,
}
);
To mint our very own NFT from the DALL-E image, we have to first create a user using the Create User API. This part is very simple as well, and can be done with a simple Node.js script.
const { OriginalClient, Environment } = require("original-sdk");
const client = new OriginalClient(
"YOUR_API_KEY",
"YOUR_SECRET_KEY",
{
env: Environment.Development,
}
);
// Returns a response object. Access the user's UID through the `data` attribute.
client
.createUser()
.then((response) => {
const userUid = response.data.uid;
console.log(userUid);
})
.catch((error) => {
console.error(error);
});
To mint an NFT, use the Create a New Asset API with DALL-E 3’s image URL and the accompanying details. Define asset parameters including user ID, client ID, collection UID, and the NFT’s details like name, image URL (from DALL-E 3), and attributes. Then, run to create the asset:
const { OriginalClient, Environment } = require("original-sdk");
const client = new OriginalClient(
"YOUR_API_KEY",
"YOUR_SECRET_KEY",
{
env: Environment.Development,
}
);
const newAssetParams = {
// User, client, and collection identifiers
user_uid: "USER_ID_FROM_CREATE_USER_API",
collection_uid: "YOUR_COLLECTION_URL",
// NFT details
data: {
name: "DALL-E Image NFT",
unique_name: true,
image_url: "YOUR_DALLE_IMAGE_URL",
store_image_on_ipfs: true,
},
};
client
.createAsset(newAssetParams)
.then((response) => {
const assetUid = response.data.uid;
console.log(assetUid);
})
.catch((error) => {
console.error(error);
});
This process turns your unique DALL-E 3 artwork into a minted NFT, ready for the digital marketplace!